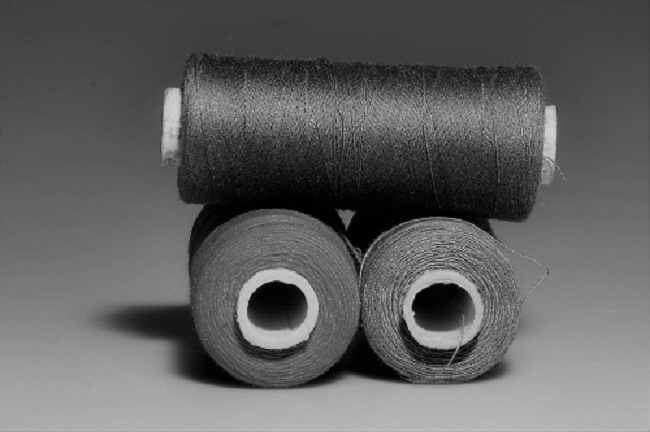
Beginner String “Theory” in JavaScript
When do I use single quotes (‘’), double quotes (“”), or backticks (``)?
Let’s start off with the easy statement – single quotes and double quotes do the same thing in JavaScript. Each of my examples will show either single quotes or double quotes – never both in the same example as the non-matching syntax will produce an error – to show that they both operate the same way.
Quotes will keep a string of text together:
Code | Displays on Console |
---|---|
console.log(“Hello, thank you for visiting!”); | Hello, thank you for visiting! |
Code | Displays on Console |
---|---|
console.log(‘10 + 2’); | 10 + 2 |
Notice how it's only the string of text that will display, not the calculated sum.
Without quotes, numbers are treated as integers in an equation:
Code | Displays on Console |
---|---|
console.log(10 + 2); | 12 |
When there are no quotes around an equation, the calculated result will display.
You can concatenate strings together:
Code | Displays on Console |
---|---|
console.log(‘abc ’ + ‘123’); | abc 123 |
Notice how the space after c is included in the display.
Taking our number example from earlier and placing each number in quotes:
Code | Displays on Console |
---|---|
console.log(“10” + “2”); | 102 |
The use of quotes around each individual number will join the contents within the quotes together.
When using constants or variables, use backticks to include the values of them in your string:
Code | Displays on Console |
---|---|
const language = “JavaScript”; console.log(`Learning ${language} is fun!`); |
Learning JavaScript is fun! |
If we were to use either double quotes or single quotes, a different display would occur:
Code | Displays on Console |
---|---|
const language = “JavaScript”; console.log("Learning ${language} is fun!"); |
Learning ${language} is fun! |
The use of quotes around the whole string will keep the contents within the quotes together.
I hope this quick post helps with how you code your strings in JS!
Racquel Tung
Racquel is a full-time working mom to two fierce little girls. She, her husband, the kiddos, and shiba inu reside in New Jersey.
Racquel is a forever a student; obtaining degrees and certificates to support her pharmaceutical career. Her latest venture, however, is one that serves her personal interest: coding. She looks forward to the challenge set forth by Moms Can & Co to be fundamentally ready as a Junior Web Developer.
Learn Digital Skills
Find out when the next cohort begins!
The most comprehensive program to up your game in the remote career world.
Learn More